Here is a simple example of how to consume a WebService in a console app with C#. For this tutorial, we will use the free service from the European commission you can use to validate tax (VAT) number.
Here is the web interface: http://ec.europa.eu/taxation_customs/vies/
First of all, create a new console application (named CheckVat) and add a new service reference.
To do that, you just need to right click on the References and select Add Service Reference:
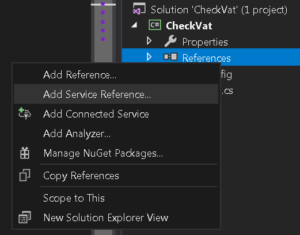
Visual Studio – add a New Service Reference
In this window, please enter the address of the service: http://ec.europa.eu/taxation_customs/vies/checkVatService.wsdl
And give it a name (Namespace): TaxService
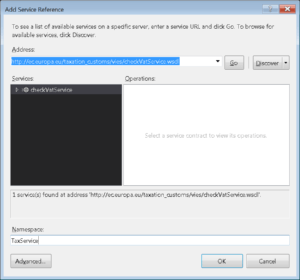
Visual Studio – define new service reference
Now you can easily call the webservice and use the method checkVat from our TaxService:
using System; namespace CheckVat { class Program { static void Main(string[] args) { try { using (var client = new TaxService.checkVatPortTypeClient()) { string name; bool valid; string address; string countryCode = "BE"; string vatNumber = "0412121524"; var result = client.checkVat(ref countryCode, ref vatNumber, out valid, out name, out address); Console.WriteLine(result); Console.WriteLine(valid ? "VALID" : "NOT VALID"); Console.WriteLine(name); Console.WriteLine(address); Console.ReadKey(); } } catch (Exception e) { Console.WriteLine(e); } } } }
Finally, here is the result of our console application, checking the VAT number for a restaurant company:
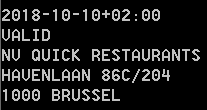
check VAT result
Respect the rules!
However, be careful. Your IP address can be banned or blacklisted from their services. Don’t spam them with wrong data or too many times with the same VAT number.
http://ec.europa.eu/taxation_customs/vies/help.html
Happy coding! 🙂