In this article, we will talk about the config file used for a C# application. What is the goal of it, and how to use it. How to get values and create your own config section.
What is a config file?
It happens sometimes (quite often actually) that we need to store some parameters, default settings, framework or external libraries values and the config has been made for that purpose.
How to use the AppSettings?
We will first try to get a variable stored in the Config File (App.config or Web.config). Let’s say here we want to store a path to write some logs:
<configuration>
...
<appSettings>
<add key="LogPath"
value="C:\temp\log\" />
</appSettings>
...
<configuration>
Code language: HTML, XML (xml)
So here, the variable name is LogPath, and the value is C:\temp\log. Now in the code, we want to get the path to store our logs from the config file:
using System.Configuration;
...
string logPath = ConfigurationManager.AppSettings["LogPath"]
Code language: C# (cs)
That was pretty easy. Now let’s do the same but for a collection.
How to create a custom config section?
Go to your config file and add a collection. Let’s say it’s a collection of Nodes. We will create a custom section in the config file called CustomConfigSection. We need first to register it in the configSections. Here is the complete config file for our simple example:
<?xml version="1.0" encoding="utf-8" ?>
<configuration>
<configSections>
<section name="CustomConfigSection"
type="ConfigFileDemo.CustomConfigSection, ConfigFileDemo" />
</configSections>
<startup>
<supportedRuntime version="v4.0" sku=".NETFramework,Version=v4.7.2" />
</startup>
<appSettings>
<add key="LogPath"
value="C:\temp\log\" />
</appSettings>
<CustomConfigSection>
<NodeList>
<node name="Node1" />
<node name="Node2" />
</NodeList>
</CustomConfigSection>
</configuration>
Code language: HTML, XML (xml)
Here, we will have to create a new class defining the structure of our Config Section called CustomConfigSection:
using System.Configuration;
namespace ConfigFileDemo
{
public class CustomConfigSectionElement : ConfigurationElement
{
[ConfigurationProperty("name", IsKey = true, IsRequired = true)]
public string Name
{
get { return (string)this["name"]; }
}
}
[ConfigurationCollection(typeof(CustomConfigSectionElement), AddItemName = "node")]
public class CustomConfigSectionCollection : ConfigurationElementCollection
{
protected override ConfigurationElement CreateNewElement()
{
return new CustomConfigSectionElement();
}
protected override object GetElementKey(ConfigurationElement element)
{
return ((CustomConfigSectionElement)element).Name;
}
}
public class CustomConfigSection : ConfigurationSection
{
[ConfigurationProperty("NodeList", IsDefaultCollection = true)]
public CustomConfigSectionCollection NodeList
{
get { return (CustomConfigSectionCollection)this["NodeList"]; }
}
}
}
Code language: C# (cs)
How to consume values from a custom config section?
Now, in the code, we can get our custom config collection from the config file:
using System;
using System.Configuration;
namespace ConfigFileDemo
{
class Program
{
static void Main(string[] args)
{
//Get a value from the AppSettings
string logPath = ConfigurationManager.AppSettings["LogPath"];
Console.WriteLine($"LogPath from the config: {logPath}");
//Get values from a custom config section
CustomConfigSection configSection = (CustomConfigSection)ConfigurationManager.GetSection("CustomConfigSection");
CustomConfigSectionCollection nodeList = configSection.NodeList;
foreach (CustomConfigSectionElement node in nodeList)
{
string nodeName = node.Name;
Console.WriteLine($"Node name from custom config section: {nodeName}");
}
Console.WriteLine("Press any key...");
Console.ReadKey();
}
}
}
Code language: C# (cs)
You should see something like this:
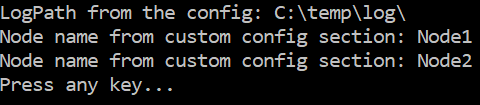
Now if you want more details about the config file, here is a link on the official Microsoft page.
Happy coding! 😉