Here is a simple example about how to create background processing in ASP.Net MVC using SQLite and Hangfire.
What is Hangfire?
Hangfire is an easy way to perform background processing in .NET and .NET Core applications. No Windows Service or separate process required. Backed by persistent storage. Open and free for commercial use.
Let me explain the three main steps to setup your own project:
Step 1 : SQLite
First of all, create your ASP.Net MVC application and install SQLite via the nuget package manager
PM> Install-Package System.Data.SQLite
Add the connection string in the web.config:
<connectionStrings> <add name="SQLiteConnection" providerName="System.Data.SQLite.EF6" connectionString="Data Source=C:\Users\User\source\repos\DemoHangfire\WebApplication\WebApplication\DataAccess\SqliteDatabase.db" /> </connectionStrings>
If you need more information about the configuration of SQLite, you can read this post.
Step 2 : Hangfire SQLite Extensions
You have to install an extension to be able to use Hangfire and SQLite. You can install directly the last version via this command in the Nuget package manager:
PM> Install-Package Hangfire.SQLite
Step 3 : Code
Finally, we can add the code in the startup.cs to configure Hangfire with SQLite:
public partial class Startup
{
public void Configuration(IAppBuilder app)
{
ConfigureAuth(app);
var options = new SQLiteStorageOptions();
GlobalConfiguration.Configuration.UseSQLiteStorage("SQLiteConnection", options);
var option = new BackgroundJobServerOptions
{
WorkerCount = Environment.ProcessorCount * 4
};
app.UseHangfireServer(option); app.UseHangfireDashboard();
app.UseHangfireServer();
RecurringJob.AddOrUpdate(() => Job(), Cron.Minutely);
}
public void Job()
{
Debug.WriteLine($"Done ! {DateTime.Now}");
}
}
Code language: C# (cs)
If you want to know more information about WorkerCount follow this link.
It’s important to know that Hangfire deals only with synchronous method (more).
Hangfire’s SQL tables will be created automatically at the first start, as explain in the documentation : “SQL Server objects are installed automatically from the SqlServerStorage
constructor by executing statements“
In this configuration, we enabled the dashboard (UseHangfireDashboard). So we can browse it via: localhost:portnumber/hanfire
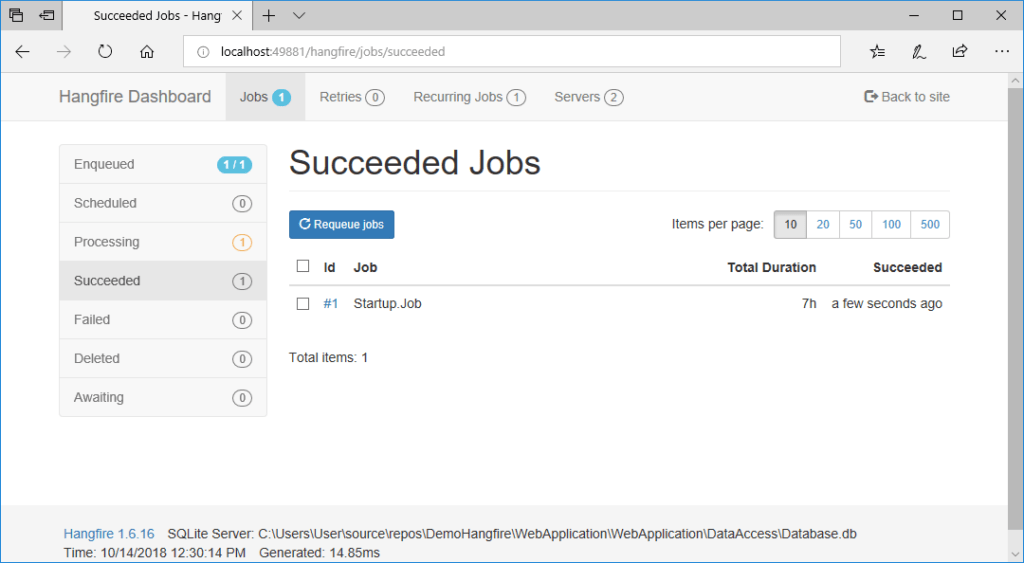
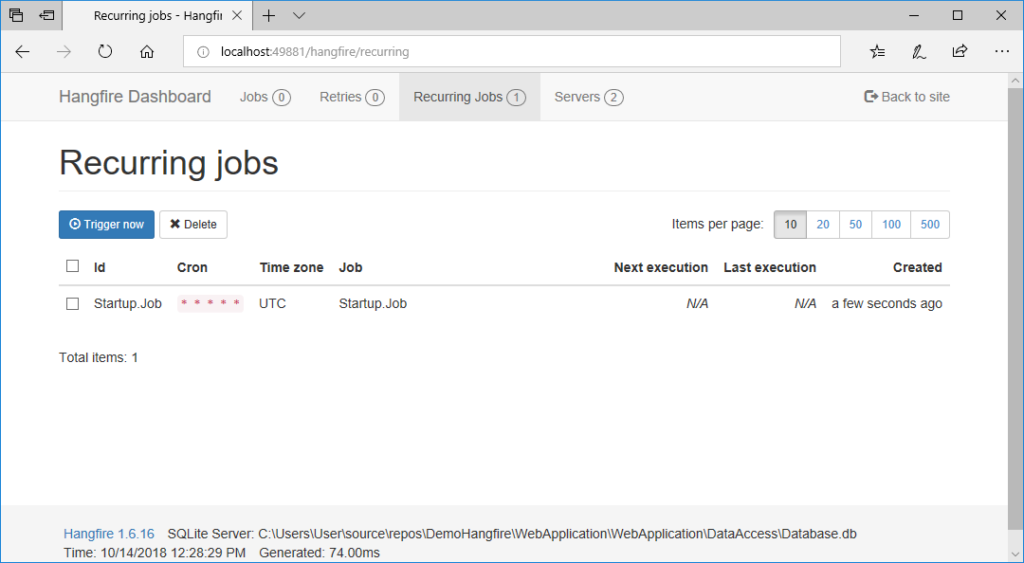
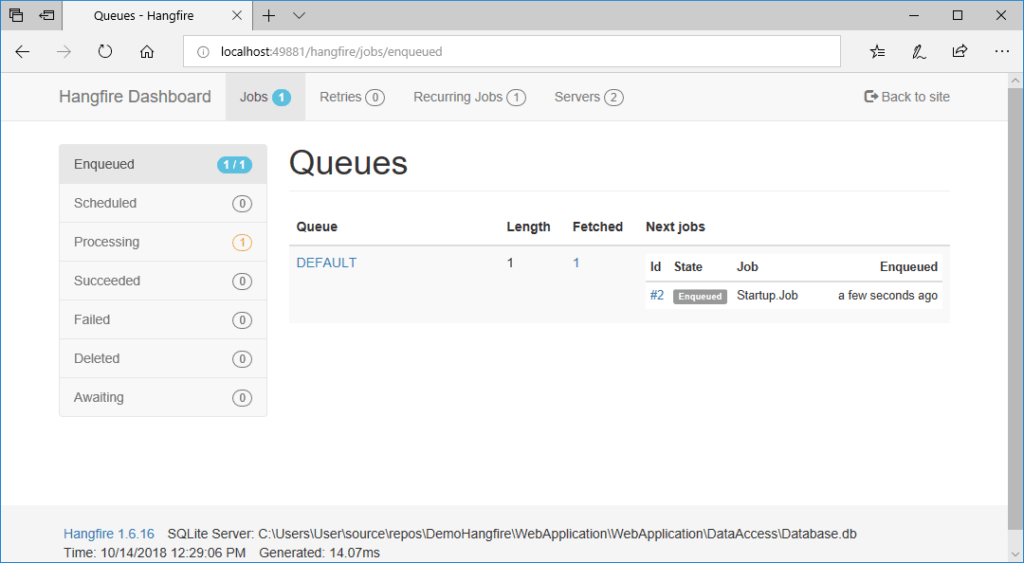
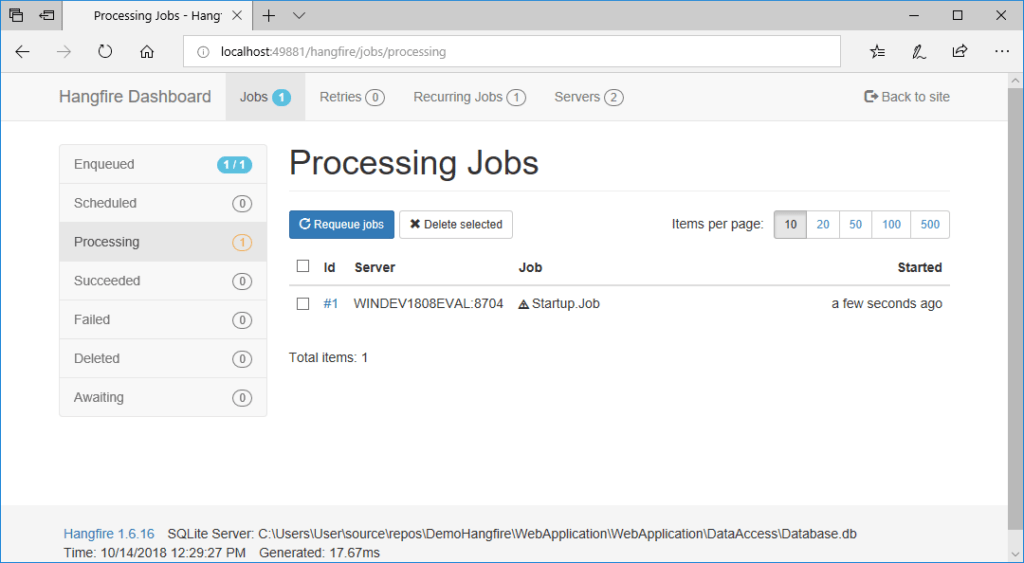