How to get current Windows 10 Theme?
In the namespace Windows.UI.ViewManagement, you can Access to the UISettings class. It will allow you to get the Window background and deduce the current theme.
public static string GetWindowThemeName()
{
var uiSettings = new UISettings();
var color = uiSettings.GetColorValue(UIColorType.Background);
if (color == Colors.White) return nameof(ElementTheme.Light);
if (color == Colors.Black) return nameof(ElementTheme.Dark);
return nameof(ElementTheme.Light);
}
Code language: C# (cs)
How to set the theme when multiple views are open?
Task.Factory.StartNew(async () =>
{
foreach (var iteratingview in CoreApplication.Views)
{
await iteratingview.Dispatcher.AwaitableRunAsync(() =>
{
if (Window.Current.Content is FrameworkElement frameworkElement)
frameworkElement.RequestedTheme = value.ToElementTheme();
}, CoreDispatcherPriority.Normal);
}
});
Code language: C# (cs)
You must iterate all the views via the CoreApplication. Secondly, you can go to the dispatcher of the view and set the RequestedTheme to the desired one.
How to set the theme to a ContentDialog or a control?
It is possible to set the theme manually in a control.
public MyContentDialog()
{
this.InitializeComponent();
RequestedTheme = Windows.UI.Xaml.ElementTheme.Light;
}
Code language: C# (cs)
How to manage theme colors in the XAML?
If you want to support different theme (e.g: Light or Dark), the best way is to use Theme Resources. You can find more information about the subject here. Brush will be adapted automatically when you change the requested theme. Here is an example of XAML code.
<TextBlock Foreground="{ThemeResource SystemBaseMediumColor}" Text="Code4Noobz"></TextBlock>
Code language: HTML, XML (xml)
In the following pictures you can compare the different render of the color between the theme.
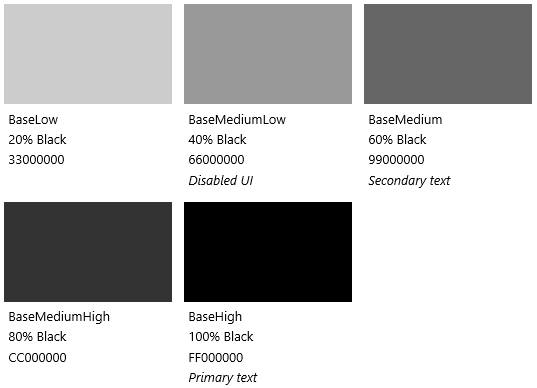
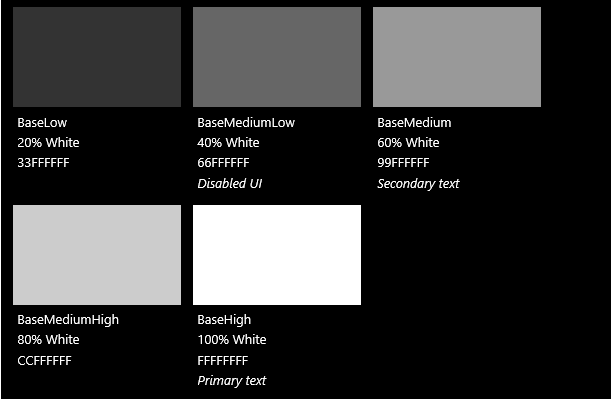
Conclusion
Finally, you will notice the importance of taking into account theming at the very beginning of you implementation. Otherwise you will be forced to cleanup all your brushes and colors used in your XAML.