Hello Devz,
Manipulating the clipboard content is pretty easy. But it can become handy when pasting it in a GridView. Here a simple example on how to paste the content of the clipboard from Excel to a GridView.
First things first! Here is how to use the clipboard:
var clipboardContent = Clipboard.GetText();
Obviously you could use the SetText(string) method as well, but it’s not the purpose in our tutorial.
What we want is to copy the content of an Excel sheet and get it in our GridView.
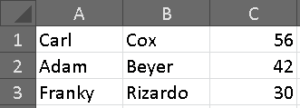
Excel content to paste to our GridView
Ok, now let’s create our WPF application, starting with the Model:
using Prism.Mvvm; namespace PasteClipboardToGridView.Models { public class Employee : BindableBase { private string _firstName; public string FirstName { get { return _firstName; } set { SetProperty(ref _firstName, value); } } private string _lastName; public string LastName { get { return _lastName; } set { SetProperty(ref _lastName, value); } } private int _age; public int Age { get { return _age; } set { SetProperty(ref _age, value); } } } }
Here is our GridView:
<Window x:Class="PasteClipboardToGridView.Views.MainWindow" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:prism="http://prismlibrary.com/" prism:ViewModelLocator.AutoWireViewModel="True" Title="{Binding Title}" Height="350" Width="525"> <Window.InputBindings> <KeyBinding x:Name="SpecialKeyPaste" Modifiers="Ctrl" Key="V" Command="{Binding PasteCommand}" /> </Window.InputBindings> <Grid> <ListView HorizontalAlignment="Left" VerticalAlignment="Top" Margin="10" Name="Employees" ItemsSource="{Binding EmployeeList}"> <ListView.View> <GridView> <GridViewColumn Header="FirstName" DisplayMemberBinding = "{Binding FirstName}"/> <GridViewColumn Header="LastName" DisplayMemberBinding="{Binding LastName}"/> <GridViewColumn Header="Age" DisplayMemberBinding="{Binding Age}"/> </GridView> </ListView.View> </ListView> </Grid> </Window>
As you can see, we added a KeyBinding to bind the CTRL+V event to a PasteCommand.
And now our ViewModel:
using PasteClipboardToGridView.Models; using Prism.Commands; using Prism.Mvvm; using System; using System.Collections.ObjectModel; using System.Linq; using System.Windows; namespace PasteClipboardToGridView.ViewModels { public class MainWindowViewModel : BindableBase { #region Properties private string _title = "Clipboard and GridView"; public string Title { get { return _title; } set { SetProperty(ref _title, value); } } private ObservableCollection<Employee> _employeeList; public ObservableCollection<Employee> EmployeeList { get { return _employeeList ?? (_employeeList = new ObservableCollection<Employee>()); } set { SetProperty(ref _employeeList, value); } } #endregion #region Commands private DelegateCommand _pasteCommand; public DelegateCommand PasteCommand => _pasteCommand ?? (_pasteCommand = new DelegateCommand(PasteClipBoard)); #endregion public MainWindowViewModel() { this.LoadEmployees(); } private void LoadEmployees() { var employee1 = new Employee { FirstName = "John", LastName = "Smith", Age = 35 }; this.EmployeeList.Add(employee1); var employee2 = new Employee { FirstName = "Anna", LastName = "Doo", Age = 37 }; this.EmployeeList.Add(employee2); var employee3 = new Employee { FirstName = "Elon", LastName = "Musk", Age = 47 }; this.EmployeeList.Add(employee3); } private void PasteClipBoard() { var clipboardContent = Clipboard.GetText(); if (string.IsNullOrEmpty(clipboardContent)) return; //Rows in Excel are splitted by Cariage Return - Line Feed ("\r\n") var rows = clipboardContent .Split(new string[] { "\r\n" }, StringSplitOptions.None) .Where(x => !string.IsNullOrEmpty(x)) .ToList(); foreach (var row in rows) { //Columns in Excel are splitted by a Tab ("\t") var columns = row.Split('\t'); if (columns.Count() < 3) { MessageBox.Show("Please select 3 columns in Excel.", "Clipboard content incorrect", MessageBoxButton.OK, MessageBoxImage.Exclamation); return; } var age = 0; int.TryParse(columns[2], out age); var employee = new Employee { FirstName = columns[0], LastName = columns[1], Age = age }; this.EmployeeList.Add(employee); } } } }
So when you copy the content of the Excel file, rows are separated by “\r\n” and columns by “\t”.
And here is the result:
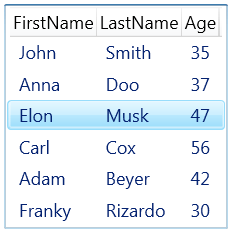
wpf copy paste from Excel to GridView result
Happy pasting! 🙂