Introduction
This post is linked to my previous one about the keyboard (available here). The idea now is to manage InputTextView size and scrolling correctly. It’s a nightmare to scroll inside a small input. That is the reason why the scrolling is disabled until the height reach the middle of the screen. In the screenshot bellow you can see a preview of your future result.
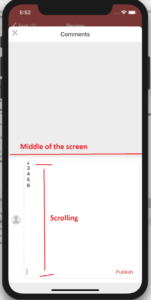
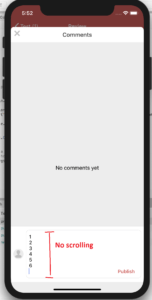
I saw different way to achieve this goal on Internet but it was always too complicated! So I propose an easy way to accomplish this behavior.
As you can see bellow we need two constraint with a name. In red, the height of the input. In green, the height of the footer view. The other part of the screen is a table view in my case, let’s call it CommentTableView.
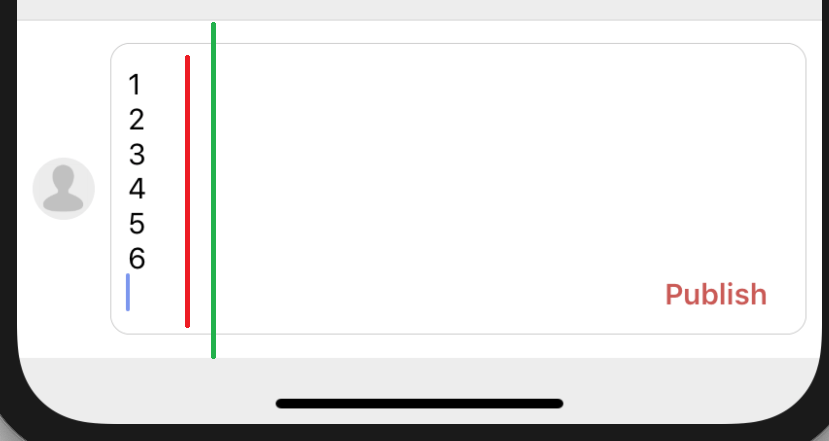
Implementation
First you should enable the scrolling because the goal is to manage InputTextView size and scrolling. Don’t forget that 😉
public override void ViewDidLoad()
{
base.ViewDidLoad();
InputTextView.ScrollEnabled = true;
Code language: C# (cs)
In a second part, we should add an observer to the InputTextView to detect when the size change. (AddObserver)
public override void ViewDidAppear(bool animated)
{
base.ViewDidAppear(animated);
AddObservers();
Code language: C# (cs)
void AddObservers()
{
InputTextView.AddObserver(this, "contentSize", NSKeyValueObservingOptions.OldNew, IntPtr.Zero);
}
Code language: C# (cs)
Here is the logic inside the method OnSizeChanged: First we define if the size of the inputTextView increase or decrease (the user writes text or deletes text). It gives us a simple Boolean, the famous isIncreasing.
When isIncreasing equals true. There is one check newValue.Height > CommentTableView.Frame.Height. It allows you to know if the rest of the screen (CommentTableView) still has a valid height (in our case we want to stay in the middle of the screen). Depending on this information, we allow the increasing of the footer or not.
When isIncreasing equals false, you update the height to the new value. In our case, the footer view (the container of the InputTextView) has always a height equals to the InputTextView plus 32 (as a custom margin).
private void OnSizeChanged(NSObservedChange nSObservedChange)
{
CGSize oldValue = ((NSValue)nSObservedChange.OldValue).CGSizeValue;
CGSize newValue = ((NSValue)nSObservedChange.NewValue).CGSizeValue;
var value = newValue.Height - oldValue.Height;
bool isIncreasing = value > 0;
if (isIncreasing)
{
if (FooterViewHeightConstraint.Constant <= CommentTableView.Frame.Height)
{
if(newValue.Height > CommentTableView.Frame.Height)
{
nfloat height = CommentTableView.Frame.Height;
InputTextViewHeightConstraint.Constant = height;
FooterViewHeightConstraint.Constant = height + 32;
}
else
{
nfloat height = newValue.Height;
InputTextViewHeightConstraint.Constant = height;
FooterViewHeightConstraint.Constant = height + 32;
CGRect frame = InputTextView.Frame;
frame.Size = InputTextView.ContentSize;
InputTextView.Frame = frame;
}
}
}
else
{
if(newValue.Height <= FooterViewHeightConstraint.Constant)
{
InputTextViewHeightConstraint.Constant = newValue.Height;
FooterViewHeightConstraint.Constant = newValue.Height + 32;
}
}
}
Code language: C# (cs)
Conclusion
Finally, you are now able to manage InputTextView size and scrolling. Nothing can stop you to implement your own chat or comment page! So let’s go!
[…] this post you will see how to implement LazyLoading on your TableView. In my previous article, I explained how to manage the scrolling. It’s time to continue our journey with Xamarin […]